Lesson 6: Random Numbers
Overview
Question of the Day: How can we make our programs behave differently each time they are run?
Students are introduced to the randomNumber()
block and how it can be used to create new behaviors in their programs. They then learn how to update variables during a program. Combining all of these skills, students draw randomized images.
Purpose
This lesson introduces randomness, which is important both as a way to make programs more interesting, but also to motivate the use of variables. In the middle of the activity, students are exposed to a variable that is updated multiple times in the program, expanding their understanding of how variables can be used.
Assessment Opportunities
1. Generate and use random numbers in a program
See level 7 in Code Studio.
2. Update a value stored in a variable
See level 5 in Code Studio. Check that students have updated the value of "petalSize" between drawing the two flowers.
Agenda
Lesson Modifications
Warm Up (5 min)
Activity (35 min)
Wrap Up (5 min)
View on Code Studio
Objectives
Students will be able to:
- Generate and use random numbers in a program
- Update a value stored in a variable
Preparation
Review the level progression in Code Studio
Links
Heads Up! Please make a copy of any documents you plan to share with students.
For the Teachers
- Random Numbers - Slides
Introduced Code
Teaching Guide
Lesson Modifications
Attention, teachers! If you are teaching virtually or in a socially-distanced classroom, please see these modifications for Unit 3.
Warm Up (5 min)
Discussion Goal
The goal of this discussion is to set context for the introduction of random numbers. Students may come up with various ideas related to user interaction or gathering input from other sources. Allow them to discuss the different ideas that they have, but eventually turn the conversation to the idea of randomness.
Prompt: So far, our programs have done the same thing every time that we run them. Are there any times that you'd want a program to do something differently each time it was run?
Discuss: Allow students time to write down some ideas, then discuss as a group.
Remarks
So far, we've wanted our programs to do exactly as we've coded, and most of our surprises have been bugs. Today we're going to look at how we can code random behaviors into our programs so that we can get some good surprises.
Question of the Day: How can we make our programs behave differently each time they are run?
Activity (35 min)
Programming Images
Transition: Move students onto Code Studio
Share: If some students have taken extra time to work on their projects, give them a chance to share their more complex rainbow snakes. Focus conversation on which parameters students are manipulating or randomizing to create their drawings.
Wrap Up (5 min)
Question of the Day: How can we make our programs behave differently each time they are run?
Discussion Goal
The discussion is intended to have students think about the wider implications of randomness in games and other programs. Although there is no block to general random data other than numbers, in later lessons students will learn techniques that will allow them to use random numbers to randomly choose from a variety of behaviors.
Prompt: So far, we've only looked at random numbers. Are there any other things that you might like to be random in your program?
Share: Allow students to share out what sorts of random things they might like in their programs.
- Lesson Overview
- 1
- Exploration
- 2
Student Instructions
Random Numbers
You have a new block in the Math
drawer called randomNumber()
. If you make your drawings with random numbers it will look a little bit different every time you run your program. You can learn about the block by hovering over it in the toolbox and choosing "See examples".
Do This
- Run the program several times to see how it works.
- Change the numbers inside
randomNumber
and run the code again a few times to see what changes.
Student Instructions
Random Numbers
Here's the same sun from last time. Right now only the x-coordinate is random, but you can make the y-coordinate random, too.
Do This
- Use
randomNumber()
for the ellipse's Y parameter so the circle is drawn in a random Y position, too. - As long as your circle is appearing at random X and Y positions, you can move on.
Student Instructions
Variables and Random Numbers
Variables can be assigned a random number too. This lets you save a single random value so that you can use it as many times as you want in your program.
Do This
- Assign a random number to the variable
eyeSize
so the the eyes automatically change size every time you run the program. Remember, the left and right eyes should always match each other!
Show me how...
var eyeSize = randomNumber(10,50);
Student Instructions
Changing variable values
These two flowers use a single variable to store their petal size, but the value stored in the variable changes in the middle of the program.
Do this
- Run the program several times to see how it works.
- Discuss the code with your partner.
- Change the program so that the flowers have random petal sizes as in the image.
Student Instructions
Try out your new skills on these levels.
Choose from the following activities:
Make the planets different sizes using random numbers.
Keep the rectangles stacked on top of each other by changing the way random numbers are used.
Student Instructions
Modify the Planets
You have learned how to randomize things, so it's time to put all the knowledge to use in this scene!
Do This
- Make this scene more fun! Give the planets random sizes so it's always changing. And for added fun, randomize their positions a little bit!
Student Instructions
Debug: Random Shape Movement
Right now in this program each rectangle has its own random position. Can you make it so that the rectangles are always stacked on top of each other, but the entire stack is randomly positioned?
Do This
- Run this program to see how it works.
- Use the variable
xPosition
to draw the bottom two rectangles at the same x position as the top pink rectangle.
- Assessment
- 7
Assessment Opportunities
Generate and use random numbers in a program, use arguments to change the way a method or command runs.
Extensive Evidence
All circles have reasonable horizontal positions, different colors, and appear at random vertical positions, and some circles have a random size.
Convincing Evidence
All circles have reasonable horizontal positions, different colors, and appear at random vertical positions
Limited Evidence
At least one student-created circle has a new color, appears at a random vertical position, and has a different horizontal position than any others.
No Evidence
No student produced circles, or any new circles share a color or horizontal position with circles produced by the original code.
Student Instructions
Rainbow Snake
This program draws a rainbow snake. To make the snake draw differently every time you'll need to use random numbers.
Do This
- Run the program several times to see how the starter code works.
- Add at least three new different colored circles to your rainbow snake.
- Use
randomNumber()
to make the rest of the snake's body move up and down like the first three.
Challenge: Can you make the size of some of the circles random, too?
Student Instructions
Try out these extra challenges with random numbers.
Choose from the following activities:
Randomize the colors of this rainbow.
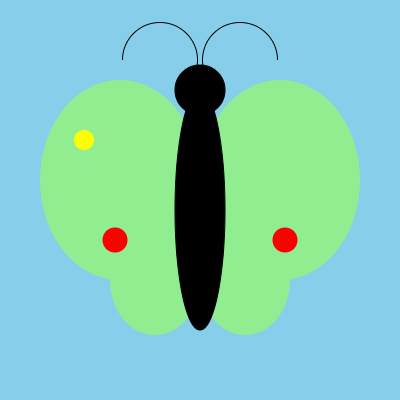
Use math to restrict the range of random numbers.
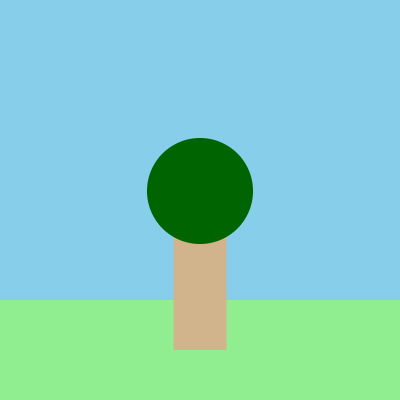
Randomize the width of the tree, but make sure the leaves are always big enough.
Randomize all the properties of these lines.
Randomize all the properties of these arcs.
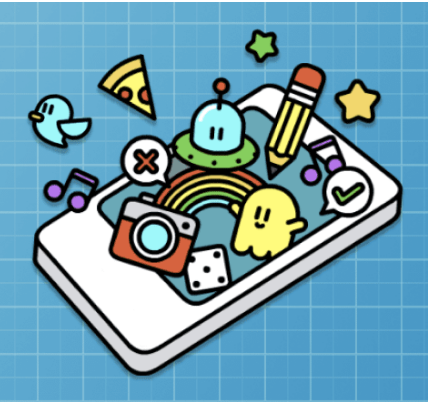
None
Student Instructions
Random RGB
You have learned how to randomize things, so it's time to put all the knowledge to use in this scene!
Do This
- Right now all the colors in this scene are set, using both rgb and random, randomize the colors here!
Student Instructions
Symmetry
You have learned how to randomize things, but what if you want two random shapes to be related in some way? Using a variable and experimenting with the math operations (+, -, *, /) can let you create effects like symmetry in your work. Butterfly wing patterns are naturally symmetrical. This drawing's red spots are placed randomly, but the second spot is always the same distance from the right side of the screen as the first spot is from the left.
Do This
- Study how the red spots are drawn (lines 24-25). Note how subtraction is used. (Show me where. )
- Use this pattern again to create a second yellow spot to give the butterfly better symmetry.
- As a challenge, create a spot3 variable, then add two more ellipses to the butterfly's wings.
Student Instructions
Randomize Two Shapes Together
Sometimes you want to randomize parts of a drawing, but still have the shapes work together. You can often do this by using variables and math operations (+, -, *, /). This tree drawing uses height
to control the height of the trunk and vertical position of the leaves. It uses width
to set the width of the trunk. A different leavesWidth
variable controls the size of the leaves.
Do This
- Notice the ranges of the variables
width
andleavesWidth
. How are they different? - Run the program repeatedly until you notice what sometimes goes wrong.
- Edit the code so that the width of the leaves is always exactly double the width of the tree trunk.
Student Instructions
Random Lines
In the scene below all it is are just a bunch of straight lines. Not very exciting.
Do This
- Make this scene more fun! You now know how to randomize things like position, but don't forget about things like stroke color and stroke weight. Have fun!
Student Instructions
Random Arcs
In the scene below all it is are just a bunch of arcs that look alike. Not very exciting.
Do This
- Make this scene more fun! You now know how to randomize things like position and angle, but don't forget about things like fill color, stroke color, and stroke weight. Have fun!
Student Instructions
Free Play
Use what you've learned to create whatever you like. When you're finished, you can click to send your creation to a friend, or
to send it to your Projects Gallery.
Standards Alignment
View full course alignment
CSTA K-12 Computer Science Standards (2017)
AP - Algorithms & Programming
- 2-AP-11 - Create clearly named variables that represent different data types and perform operations on their values.
- 2-AP-13 - Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
- 2-AP-17 - Systematically test and refine programs using a range of test cases.
- 2-AP-19 - Document programs in order to make them easier to follow, test, and debug.