Lesson 22: Collisions
Overview
Question of the Day: How can programmers build on abstractions to create further abstractions?
In this lessson, students program their sprites to interact in new ways. After a brief review of how they used the isTouching
block, students brainstorm other ways that two sprites could interact. They then use isTouching
to make one sprite push another across the screen before practicing with the four collision blocks (collide
, displace
, bounce
, and bounceOff
).
Purpose
This lesson introduces collisions, another useful abstraction that will allow students to manipulate their sprites in entirely new ways. While students could theoretically have written their own displace, collide, or bounce commands, the ability to ignore the details of this code allows them to focus their attention on the high level structure of the games they want to build.
This lesson is also intended to give students more practice using the new commands they have learned in the second chapter. This the last time they will learn a new sprite behavior, and following this lesson students will transition to focusing on how they organize their increasingly complex code.
Assessment Opportunities
-
Model different types of interactions between sprites.
See Level 8 in Code Studio.
-
Describe how abstractions can be built upon to develop even further abstractions
In the wrap up, make sure students understand that blocks such as
isTouching
hide the complexity or details of the code inside, allowing them to tackle more complex problems.
Agenda
Lesson Modifications
Warm Up (5 min)
Activity (30 min)
Wrap Up (10 min)
View on Code Studio
Objectives
Students will be able to:
- Model different types of interactions between sprites.
- Describe how abstractions can be built upon to develop even further abstractions
Links
Heads Up! Please make a copy of any documents you plan to share with students.
For the Teachers
- Collisions - Slides
Vocabulary
- Abstraction - a simplified representation of something more complex. Abstractions allow you to hide details to help you manage complexity, focus on relevant concepts, and reason about problems at a higher level.
Introduced Code
Teaching Guide
Lesson Modifications
Attention, teachers! If you are teaching virtually or in a socially-distanced classroom, please see these modifications for Unit 3.
Warm Up (5 min)
Discussion Goal
Goal: The goal of this discussion is for students to brainstorm ways to solve the problem of having one sprite push another across the screen. There's no need for students to come to a consensus, because they will each have a chance to try out a solution in the next level in Code Studio. Students should understand that it is possible to use blocks to produce the desired movement just with the blocks that they have already learned.
Display: Display the animated image. It is also available as a level in code studio.
Prompt: Using the blocks we already know how to use, how could we create the sprite interaction we can see in this program?
Share: Allow students to share out their ideas.
Teaching Tip
Students have seen this vocabulary before, but given its importance to the chapter, it is introduced again here.
Remarks
The first part of the problem is figuring out when the two sprites are touching, but we already figured out how to do that and can now use the isTouching
block. That means we don’t need to think about those details anymore. Using abstraction to hide the complicated details in that part of the problem means we can focus on the new part.
Vocabulary Review:
- abstraction - a simplified representation of something more complex
Question of the Day: How can programmers build on abstractions to create further abstractions?
Activity (30 min)
Assessment Opportunity
Make sure students talk about the importance of higher-level blocks, such as isTouching, and that while these blocks don't provide new functionality, hiding the complexity of the code inside of a single block allows them to tackle more complex problems.
This is also a good time to call out how far the students have progressed in their skills since the beginning of the unit. This problem would have seemed almost impossible at the beginning of the year. Some things that made the problem easier to solve were:
- Preparation: The students brainstormed and thought about solutions before trying out their code.
- Cooperation: Students worked as a group to come up with a solution
- Abstraction: Students were able to use the
isTouching
andvelocityY
blocks to hide part of the solution's complexity.
Prompt: This was a challenging problem, but we were able to solve it. What helped us to solve this problem?
Remarks
All of these things are very important, and they come up in Computer Science a lot. One thing that was particularly helpful was the isTouching
block, which hid the complicated code that tells us whether the two sprites are touching. There's also a displace
block that hides the code we just wrote, and some other blocks that hide the code for some other types of sprite interactions. You'll have a chance to try out these blocks in the next few levels.
Wrap Up (10 min)
Assessment Opportunity
Students should understand that these two blocks represent partial solutions to the problem of one sprite pushing the other, and that by hiding the details of those partial solutions, they can more easily focus on how to fit those partial solutions together to solve larger and more complex problems.
Question of the Day: How can programmers build on abstractions to create further abstractions?
Vocabulary Review:
- abstraction - a simplified representation of something more complex
Prompt: How did having the isTouching
block and the velocityX
block make it easier to solve the problem of one sprite pushing another?
- Lesson Overview
- 1
- Code Prediction
- 2
Student Instructions
Sprite Interactions
So far you've been able to create simple sprite interactions by using the sprite.isTouching()
block. For example, you've reset a coin to a different location on the screen when a character touches it. Now it's time to start making sprites have more complex interactions.
Do This
- Run the program and observe the interaction between the two sprites.
- Discuss with a neighbor: Using only the commands you already know how could you create this kind of interaction? There are many ways to do it, but here are some blocks to consider:
Be ready to share your ideas with your classmates.
Student Instructions
Program a Sprite Interaction
Here's a similar program, but it doesn't work yet.
Do This
- Use your plan to program the giraffe to push the monkey off the screen.
Student Instructions
Write Your Own Sprite Interaction
Now the elephant should push the hippo off the screen. Notice that the elephant moves at a random Y velocity each time the program runs.
Do This
- Run the program to see how it works.
- Using the patterns from the last level, write code that makes the elephant push the hippo off the screen.
Student Instructions
Displace
This type of sprite interaction is so common that there's a block for it: sprite.displace()
. Someone tried to use the sprite.displace()
block to make the elephant push the hippo, but there is a bug.
Do This
- Find the line of code where the
sprite.displace()
block is used and fix the error.
Student Instructions
More Collision Blocks
Three new types of sprite interactions have been added to the toolbox, sprite.collide()
, sprite.bounce()
, and sprite.bounceOff()
. How do you think they'll affect the sprites?
Do This
- Switch out the displace block for the
sprite.collide()
,sprite.bounce()
, andsprite.bounceOff()
blocks. ( Show me where )- Hint: If you're having trouble doing this with blocks then switch over to text mode.
- Discuss with a neighbor: What is the difference between the four different sprite interactions? What do you think the purpose of each block is?
Student Instructions
Practice using the new collisions blocks.
Choose from the following activities:
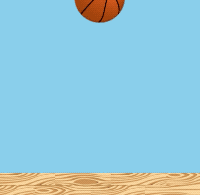
Make the basketball interact with the wooden floor.
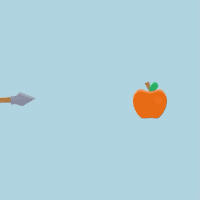
Make the arrow stop at the apple.
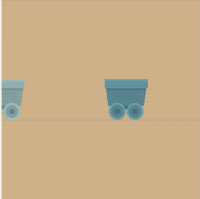
Make one cart push the next.
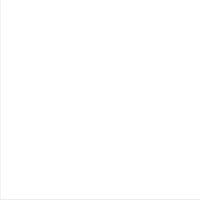
Help her push the suitcase.
Student Instructions
Collision Types
There are four types of collisions that we use in Game Lab: displace
, collide
, bounce
, and bounceOff
. These blocks will cause a certain type of interaction between the sprite and its target.
Do This
- Choose the best block to model the basketball's interaction with the floor. ( Show me where )
*Hint: You can try the different blocks out, or read more about them in the "Help and Tips" tab.
Student Instructions
Collision Types
There are four types of collisions that we use in Game Lab: displace
, collide
, bounce
, and bounceOff
. These blocks will cause a certain type of interaction between the sprite and its target.
Do This
- Choose the best block to make the arrow stop when it reaches the apple. ( Show me where )
*Hint: You can try the different blocks out, or read more about them in the "Help and Tips" tab.
Student Instructions
Collision Types
There are four types of collisions that we use in Game Lab: displace
, collide
, bounce
, and bounceOff
. These blocks will cause a certain type of interaction between the sprite and its target.
Do This
- Choose the best block to make the gray minecart stop and push the second cart. ( Show me where )
Hint: You can try the different blocks out, or read more about them in the "Help and Tips" tab.
Student Instructions
Collision Types
There are four types of collisions that we use in Game Lab: displace
, collide
, bounce
, and bounceOff
. These blocks will cause a certain type of interaction between the sprite and its target.
Do This
- Choose the best block to make the child push the suitcase. ( Show me where )
Hint: You can try the different blocks out, or read more about them in the "Help and Tips" tab.
- Assessment
- 8
Assessment Opportunities
Model complex movement on a coordinate plane.
Extensive Evidence
The program runs exactly as in the target image, with all sprite interactions shown, and there are no errors in the code.
Convincing Evidence
Multiple sprite interactions are included in the program, and at least two are as shown in the target image.
Limited Evidence
There is at least one sprite interaction, but it may not be as shown in the target image.
No Evidence
No sprite interactions are in the program.
Student Instructions
Sprite Interactions
You've now seen four different types of sprite interactions. You'll need them all for this challenge.
Do this
- For each pair of animals, choose the sprite interaction that matches the target image on the right.
Student Instructions
Try out these new blocks and challenges with collisions.
Choose from the following activities:
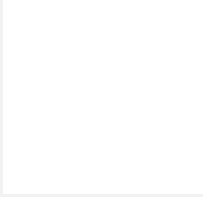
Set a sprite's debug property to understand how it bounces.
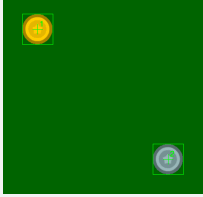
Learn how to change how sprites interact based on their collider shape.
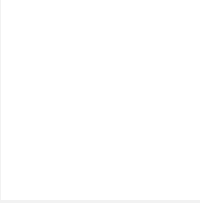
Control how much sprites bounce when they interact.
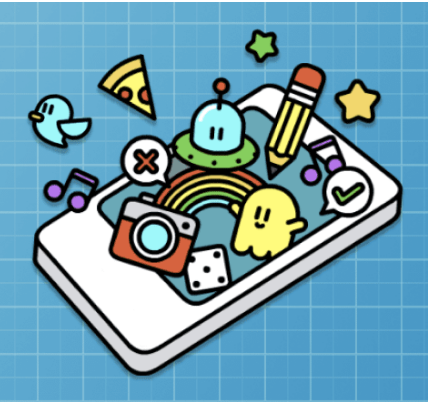
None
Student Instructions
Debug
Sometimes sprites will behave in ways that are unexpected. There is a special sprite.debug
property you can use to better understand why the sprites interact the way that they do.
Do This
These two coins are round, so you would expect them to bounce in a certain way. Something weird is happening though!
- Run the code and watch the way that the coins interact.
- Use the
sprite.debug
block to make debug 'true' for both the sprites and run the code again. - Change the gold coin's starting x position to 51 and run the code again.
- Discuss with a partner: Why do you think the coins are bouncing strangely?
Student Instructions
setCollider
Sprites interact based on the size and shape of their collider, not the images that are assigned to them. You can only see the collider when debug mode is turned on. You can change the shape of the collider using the sprite.setCollider()
block, which lets you pick between a "rectangle" or a "circle". By default all colliders are "rectangle".
Do This
- Find the
sprite.setCollider()
block for the gold coin, and change it from "rectangle" to "circle". - Add a new
sprite.setCollider()
block for the silver coin, and choose "circle" for the shape of the collider. - Run the code again to see how the sprites bounce.
Student Instructions
Bounciness
So far, bounceOff
has made sprites bounce away from other objects as fast as they bounced into them. In the real world, almost everything slows down just a little bit when it bounces off something else. You can use the bounciness
block to tell your sprite how much to slow down or speed up when it bounces off something else.
Do This
- Read the code below and press "Run" to see the behavior of the basketball and pool ball.
- Use a
bounciness
block to set the bounciness of your soccer ball. - Run the code again to see how the sprites bounce off the floor.
Student Instructions
Free Play
Use what you've learned to create whatever you like. When you're finished, you can click to send your creation to a friend, or
to send it to your Projects Gallery.
Standards Alignment
View full course alignment
CSTA K-12 Computer Science Standards (2017)
AP - Algorithms & Programming
- 2-AP-11 - Create clearly named variables that represent different data types and perform operations on their values.
- 2-AP-12 - Design and iteratively develop programs that combine control structures, including nested loops and compound conditionals.
- 2-AP-13 - Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
- 2-AP-16 - Incorporate existing code, media, and libraries into original programs, and give attribution.
- 2-AP-17 - Systematically test and refine programs using a range of test cases.
- 2-AP-19 - Document programs in order to make them easier to follow, test, and debug.