Lesson 18: Velocity
Overview
Question of the Day: How can programming languages hide complicated patterns so that it is easier to program?
After a brief review of how they used the counter pattern to move sprites in previous lessons, students are introduced to the idea of hiding those patterns in a single block. Students then head to Code Studio to try out new blocks that set a sprite's velocity directly, and look at various ways that they are able to code more complex behaviors in their sprites.
Purpose
This lesson launches a major theme of the chapter: that complex behavior can be represented in simpler ways to make it easier to write and reason about code.
In this lesson students are taught to use the velocity blocks to simplify the code for moving a sprite across the screen. This marks a shift in how new blocks are introduced. Whereas previously blocks were presented as enabling completely new behaviors, they are now presented as simplifying code students could have written with the blocks previously available. Over the next several lessons, students will see how this method of managing complexity allows them to produce more interesting sprite behaviors.
Assessment Opportunities
-
Use the velocity and rotationSpeed blocks to create and change sprite movements
See Level 10 in Code Studio. (Level 6 can be used to check rotationSpeed.)
-
Describe the advantages of simplifying code by using higher level blocks
In the wrap up, check students' descriptions of the blocks that they would create and why they would want to create them.
Agenda
Lesson Modifications
Warm Up (5 min)
Activity (30 min)
Wrap Up (5 min)
View on Code Studio
Objectives
Students will be able to:
- Use the velocity and rotationSpeed blocks to create and change sprite movements
- Describe the advantages of simplifying code by using higher level blocks
Links
Heads Up! Please make a copy of any documents you plan to share with students.
For the Teachers
- Velocity - Slides
Introduced Code
Teaching Guide
Lesson Modifications
Attention, teachers! If you are teaching virtually or in a socially-distanced classroom, please see these modifications for Unit 3.
Warm Up (5 min)
Demonstrate: Ask for a volunteer to come to the front of the class and act as your "sprite". Say that you will be giving directions to the sprite as though you're a Game Lab program.
When your student is ready, face them so that they have some space in front of them and ask them to "Move forward by 1". They should take one step forward. Then repeat the command several times, each time waiting for the student to move forward by 1 step. You should aim for the repetitiveness of these instructions to be clear. After your student has completed this activity, have them come back to where they started. This time repeat the demonstration but asking the student to "Move forward by 2" and have the student take 2 steps each time. Once the student has done this multiple times ask the class to give them a round of applause and invite them back to their seat.
Prompt: I was just giving instructions to my "sprite", but they seemed to get pretty repetitive. How could I have simplified my instructions?
Discussion Goal
Goal: The earlier demonstration should have reinforced the fact that repeatedly giving the same instruction is something you would never do in real life. You would instead come up with a way to capture that the instruction should be repeated, like "keep moving forward by 1."
Discuss: Give students a minute to write down thoughts before inviting them to share with a neighbor. Then have the class share their thoughts. You may wish to write their ideas on the board.
Remarks
Programming languages also have ways to simplify things for us. Today, we're going to look at some blocks in Game Lab that hide complicated coding patterns to make things easier for programmers.
Question of the Day: How can programming languages hide complicated patterns so that it is easier to program?
Activity (30 min)
Remarks
One way to simplify these instructions is to just tell our "sprite" to keep moving by 1 or 2, or however many steps we want. As humans, this would make instructions easier to understand, and as we're about to see there's a similar way to make our code simpler as well.
Transition: Move students to Code Studio
Circulate: These levels introduce the velocityX, velocityY, and rotationSpeed properties that you just discussed with students. Check in with students to see how they are doing and keep track of when everyone has made it to the end of level 10.
Wrap Up (5 min)
Journal
Assessment Opportunity
As students describe the blocks that they would make, ensure that they are relating the specific code that a block would use to the higher-level concept of what it would do. For example, a "velocity" block would move a sprite across the screen, and it would include blocks that use the counter pattern on a position property.
Students should describe the advantages of having these blocks, such as not needing to re-write the code all the time, or making it easier to read what the program is doing.
Prompt: You learned a few new blocks today. At first glance, these blocks did the same sorts of things we'd already done with the counter pattern, but made it simpler for us to do them. As you went through the puzzles, though, you started doing some interesting movements that we hadn't been able to do before.
- Describe one of those movements, and how you made it.
- Describe another block that you'd like to have.
- What would you name it?
- What would it do?
- What code would it hide inside?
- How would it help you?
Remarks
All of the movements that we did today are possible without the new blocks, but it would be very complicated to code them. One of the benefits of blocks like velocity is that when we don't have to worry about the details of simple movements and actions, we can use that extra brainpower to solve more complicated problems. As you build up your side scroller game, we'll keep looking at new blocks that make things simpler, so we can build more and more complicated games.
- Lesson Overview
- 1
- Skill Building
- 2
Student Instructions
velocityX
One way to move sprites in Game Lab is with the counter pattern. For example sprite1.x = sprite1.x + 1
moves a sprite by 1 pixel each frame of the draw loop. This pattern is so common that sprites have a velocityX
property that does this for you.
Do This
- Drag a
sprite.velocityX
block directly below where your sprite is created. ( Show me where ) - Write the name of your sprite in the block.
- Assign the
velocityX
property a value of 1. - Run the code. What happens?
- Re-run the code giving the
velocityX
property a different value. What's changing?
- Video: Velocity
- 3
Teaching Tip
Discussion Goals
It may not be obvious to students why the velocity block is so powerful. The immediate answer is that the velocity block allows a programmer to set the velocity at the beginning of the program and not have to worry about the counter pattern inside the draw loop (as Game Lab will take care of that). If students are having trouble of thinking of situations in which the velocity block provides a big advantage, assure them that they will tackle some problems in the coming lesson that they will need this block for.
As students give you examples, try to elicit answers that use both positive and negative numbers, and that use the x and y positions as well as sprite rotation.
Student Instructions
Questions to Consider
- Why might you want to use a velocity block instead of the counter pattern?
- Give an example of a counter pattern and how you could use a velocity block instead.
Student Instructions
Moving Down
Here is a feather sprite that should be floating down the screen. If velocityX
makes a sprite move to the right, can you find the block that will make the feather move down?
Do This
Find the block that will make the feather sprite go down the screen, and use it outside the draw loop. ( Show me where )
Student Instructions
rotationSpeed
You can use rotationSpeed
to make your sprites spin. If you want your sun to rotate by two degrees each time it's drawn, you can use sun.rotationSpeed = 2
before the draw loop, after you create your sprite.
Do This
Make the sun rotate by 3 degrees each time using the rotationSpeed
block. ( Show me where )
Teaching Tip
Inside versus outside the draw loop
This is a good time to remind students that code outside the draw loop is used to set up the program. It is for how you want your program to start. Code inside the draw loop is for things that are changing as the program is running, user interaction.
There may be some confusion that the new blocks are animation (changing position) and yet have gone outside the draw loop up until this point. That is because up until this point, the velocity has been set at the beginning of the program and not changed. When students want the velocity to change during the program, it will need to go inside the draw loop.
Student Instructions
Controlling Speed
You used rotationSpeed
outside the draw loop to make your sprite rotate when your program started. You can also use rotationSpeed
inside the draw loop to change the speed of the sprite during the game. For example, a sprite can start rotating when the user presses the space bar, and it will keep rotating until it's told to stop.
Do This
- Look at the
if
statement inside the draw loop that checks whether the space bar has been pressed. ( Show me where ) - Use the
rotationSpeed
block to make the color wheel start spinning when the user presses the space bar.
Student Instructions
Changing Velocity with Position
One advantage to using the velocity blocks inside conditionals (if
blocks) is that your sprite keeps moving, even after the condition stops being true. For example, you only had to press a key once to start the color wheel spinning, and it kept spinning forever. The code below uses if statements to make a fish sprite move in different directions.
Do This
- Look at
if
statements that check the sprite's position and set its velocity. - With your partner, discuss what you think the code will do, and write your answer below.
- Once you have submitted your answer, run the code.
Student Instructions
Back and Forth
This ball bounces back when it hits the bottom of the screen. Can you make it bounce back when it hits the top of the screen?
Do this
- Run the code and see how it works.
- Look at how conditionals and velocity are used to make the ball bounce at the bottom of the screeen.
- Add code to make the ball bounce at the top of the screen.
Student Instructions
Practice using the velocity blocks with these activities.
Choose from the following activities:
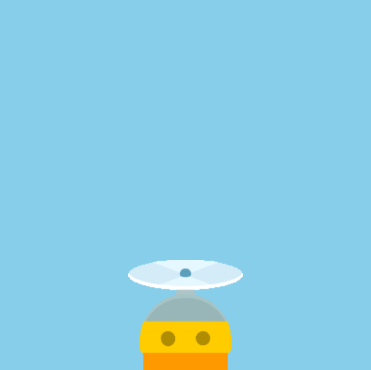
Make the robot fly once the space bar has been pressed at least once.
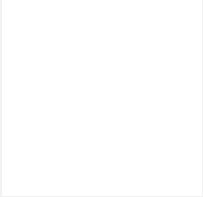
Dip the paint brush in the paint.
Student Instructions
Controlling Speed
For this animation, you'll help the "Flybot" to take off. It should start moving up when the space bar is pressed, and it should continue moving up even after the space bar is released.
Do This
Student Instructions
Paintbrush
Dip the paintbrush in the paint.
Do This
- Use a conditional to send the paint brush down if the down arrow is pressed.
- Use a different conditional to send the paint brush up if it reaches the palette.
- Hint: You will need to check its y property.
- Assessment
- 10
Assessment Opportunities
Use conditionals to control the flow of a program; model two dimensional movement on a coordinate plane.
Extensive Evidence
The fish swims to the right side of the screen, turns and swims to the left side of the screen, then repeats. The sprite appears to face the appropriate direction at all times. There are no extra blocks in the program, and the counter pattern is not used.
Convincing Evidence
The fish swims to the right side of the screen, turns and swims to the left side of the screen, but perhaps does not face the right direction while moving. The counter pattern is not used.
Limited Evidence
The fish swims to the right side of the screen, turns and swims to the left side of the screen, but does not repeat. The student only changed its velocity by using the provided first conditional statement.
No Evidence
The fish just moves right across the screen, then disappears.
Student Instructions
Swimming Right and Left
The code below should make the fish start moving right as soon as you press "Run". Using conditional statements and the .velocity
block, you can make it continually swim back and forth.
Do This
-
Look at the three
if
statements inside the draw loop. -
Use a
sprite.velocityX
block inside eachif
statement to make the three following movements: - If the user presses the right arrow key, move the fish to the right.
- If the fish gets to the right-hand side of the screen, move the fish to the left.
- If the fish gets to the left-hand side of the screen, stop the fish.
Student Instructions
Try out these new blocks and challenges with velocity.
Choose from the following activities:
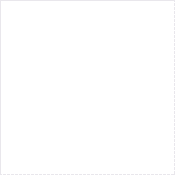
Can you change velocities four separate times?
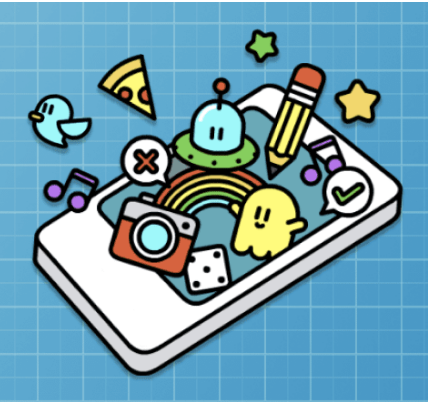
None
Student Instructions
Changing Course
Study the animation to the right. Notice that the purple alien sprite changes between x and y velocities when it is near each corner of the screen.
Do This
- Run the program and to understand how it works so far.
- Add
.velocityX
and.velocityY
blocks to each conditional to make the alien complete the full circuit.
Be careful! If the sprite starts moving diagonally, it might mean it has both an x and y velocity. In the first corner the alien needs to stop moving up and start moving right.
Student Instructions
Free Play
Use what you've learned to create whatever you like. When you're finished, you can click to send your creation to a friend, or
to send it to your Projects Gallery.
Standards Alignment
View full course alignment
CSTA K-12 Computer Science Standards (2017)
AP - Algorithms & Programming
- 2-AP-11 - Create clearly named variables that represent different data types and perform operations on their values.
- 2-AP-12 - Design and iteratively develop programs that combine control structures, including nested loops and compound conditionals.
- 2-AP-13 - Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
- 2-AP-16 - Incorporate existing code, media, and libraries into original programs, and give attribution.
- 2-AP-17 - Systematically test and refine programs using a range of test cases.
- 2-AP-19 - Document programs in order to make them easier to follow, test, and debug.