Lesson 8: Boolean Expressions and "if" Statements
Overview
In this lesson, students write if
and if-else
statements in JavaScript for the first time. The concepts of conditional execution should carry over from the previous lesson, leaving this lesson to get into the nitty gritty details of writing working code. Students will write code in a series of "toy" problems setup for them in App Lab that require students to do everything from debug common problems, write simple programs that output to the console, or implement the conditional logic into an existing app or game, like "Password Checker" or a simple Dice Game. The lesson ends with a problem requiring nested if
statements to foreshadow the next lesson.
Purpose
The main purpose here is Practice, Practice, Practice. The lesson asks students to write if-statements in a variety of contexts and across a variety of program types and problem solving scenarios.
Agenda
Getting Started (5 Minutes)
Activity (80 Minutes)
Wrap-up (10 Minutes)
View on Code Studio
Objectives
Students will be able to:
- Write and test conditional expressions using comparison operations
- Given an English description write code (if statements) to create desired program logic
- Use the comparison operators (<, >, <=, >=, ==, !=) to implement decision logic in a program.
- When given starting code add if, if-else, or nested if statements to express desired program logic
Preparation
- Forum
- Review student instructions in Code Studio (see below) along with teacher commentary.
- (Optional) A copy of (Optional) Flowcharts - Activity Guide
- (Optional) (Optional) Flowcharts Key
Links
Heads Up! Please make a copy of any documents you plan to share with students.
For the Students
- (Optional) Flowcharts - Activity Guide
Vocabulary
- Boolean - A single value of either TRUE or FALSE
- Boolean Expression - in programming, an expression that evaluates to True or False.
- Conditionals - Statements that only run when certain conditions are true.
- If-Statement - The common programming structure that implements "conditional statements".
- Selection - A generic term for a type of programming statement (usually an if-statement) that uses a Boolean condition to determine, or select, whether or not to run a certain block of statements.
Introduced Code
Teaching Guide
Getting Started (5 Minutes)
When vs. If
Teaching Tip
These are nuanced distinctions and may not be immediately clear to every student. As students gain more experience with if statements, the difference between events and if statements will likely become more clear. For now, they should at the very least understand there is a difference between the two and begin to get in the habit of asking whether they want to run a block of code based on a user action, a condition, or some combination of the two.
Remarks
In everyday conversation, it is common to interchange the words “when” and “if,” as in “If the user presses the button, execute this function.” The English language is tricky. We often say “if” the button is clicked when really we mean “when” a button is clicked. This can cause confusion because “if” has a well-defined meaning in programming.
How are conditionals (if statements) different from events?
Here is one way to think about it:
- Events are setup by a programmer, but triggered by the computer at any momement in time.
- If statements are a way a programmer can have her code make a decision during the normal flow of execution to do one thing or another.
As we have already seen in prior lessons, an if statement is evaluated when the code reaches a particular line and uses a true/false condition (like a comparison between values e.g., score == 5), to decide whether to execute a block of code.
Transition
- As we begin to write event-driven programs with if-statements we need to be clear about what we mean, or what we intend our programs to do.
- Sometimes when you say "if" you mean "when" and vice-versa. Just be on the lookout.
Optional: Flow Charts
Some people find flow-charting a useful exercise for thinking about if-statements.
Here is an optional activity: (Optional) Flowcharts - Activity Guide you can do with your students to warm up on paper.
Alternatively, you might revisit this activity after students have had some experience writing if-statements to solidify their understanding.
Activity (80 Minutes)
App Lab: Boolean expressions and if-statements
Transition to Code Studio
Students will use be introduced to conditionals by solving many different types of small puzzles and writing many small programs in different contexts with different kinds of output.
Read the student instructions and teacher commentary for more info.
Wrap-up (10 Minutes)
Compare and Contrast - easy/hard
Discussion Goal
There are of course no right answers to these prompts. But it should be an opportunity for students give voice to new learnings or frustrations.
It's also an opportunity for you get insight about areas where your students are struggling, or things you might need to revisit.
Reflection Prompt:
-
"You've now had experience reasoning about if-statements on paper with the "Will it Crash?" activity, and now actually writing if-statements in working code. Compare and Contrast these experiences.
- For "Will it Crash" - what was easy? what was hard?
- For this lessson, writing if-statements - what was easy, what was hard?
- If there was one thing you wish you understood better at this point, what would it be?
Nested if statements
Prompt:
- "The last problem ("it's the weekend") was tricky. What made it hard? How did you end up solving it?"
Let students discuss for a moment and then bring to full class discussion. Points to raise:
- What made it hard was that you needed to check more than one condition at the same time. You needed to say "it's saturday OR sunday". That's more than one condition to check.
- So a solution (using only what we know so far) is to nest if-statements.
- Nesting if statements is one way to check more than one condition at a time.
Transition
There are other ways to check more than one condition at a time that we will learn about in the next lesson.
- Lesson Overview
- Student Overview
- Introduction to Conditionals: Boolean Expressions
- 2
Student Instructions
Points to pay attention to:
- What's a Boolean Expression?
- Why is it called a "Boolean"?
- Is this a legal expression?
10 > 25
?
- Boolean Expressions and Comparison Operators
- 3
Student Instructions
Word Soup: Conditionals, Booleans, expressions, statements
Boolean, Boolean values, Boolean expressions:
- A Boolean value is simply a computer science-y term that means a true/false value.
- A Boolean expression is a statement that evaluates to a Boolean value (a single true/false).
Condition, Conditionals, Conditional Statements:
- "Conditional" is simply a generic term for code that alters program flow based on true/false values (like an
if
statement) - Examples: Condition, Conditionals, Conditional statements, conditional execution
Comparison Operators
A common type of condition to check is a comparison of two values. Here are 6 common comparison operators. Each compares a value on the left with a value on the right and returns a Boolean value -- true or false. Most of these do what you would expect.
Why these symbols: ==, !=, <=, and >=?
-
We use
==
because the single equal sign=
is the assignment operator. We need something different to indicate we want to compare two values instead of assign one to the other.Common mistake: writing something like
if (age = 18)
instead ofif (age == 18)
. We'll make sure we get this down later. -
We use
!=
,<=
, and>=
because they only requires ASCII symbols. Historically the mathematical symbols≠
,≤
and≥
were hard or impossible to produce on some systems. The!
is universally read as "not".
Reference: Examples
Below are a bunch of examples of how you might see comparisons in code. Review them if you like or continue on and come back if you need reference.
Compares two values - numbers, strings, or other booleans - and returns true if they are equal, otherwise false.
"Hello" == "hello"
returns false -- because the strings are slightly different."3" == 3
returns true --==
tries to be forgiving. If it can "coerce" a string into a number it will do so to compare. 1(2+1) == 3
returns true -- because the arithmetic expression evaluates to 3.x == 7
returns true -- when the variable x has the value 7.
1. While it is a useful feature that == will coerce a string into a number, it is considered TRICKY since string "3" is not the same as the integer 3 underneath the hood. There are circumstances when you would consider these not equal. There is a "strict" equality operator - the "triple equal" ===
which makes sure that the both the type of data and value are equal. So "3" === 3
is false.
Compares two values - numbers, strings, or other booleans - and returns true
if they are not equal, otherwise false
.
"Hello" != "hello"
returns true -- because the strings are slightly different."3" != 3
returns false -- because the string 3 can be coerced into a number before comparing with 3. (see notes above about the forgiving ==).(2+1) != 3
returns false -- because the arithmetic expression evaluates to 3.x != 7
returns true -- when the variable x is any value other than 7.
Compares two values to see if the number on the left is greater than the number on the right.
4 > 3
returns true3 > 7
returns falseage > 17
returns true -- when the value of the variable "age" is strictly greater than 17, otherwise false.
Compares two values to see if the number on the left is less than the number on the right.
4 < 3
returns false3 < 7
returns trueage < 17
returns true -- when the value of the variable "age" is strictly less than 17, otherwise false.
Compares two values to see if the number on the left is less than or equal to the number on the right.
3 <= 4
returns true4 <= 3
returns falseage <= 18
returns true -- when the value of the variable "age" is 18 or less.
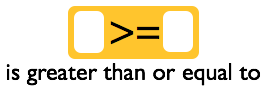
3 >= 4
returns false4 >= 3
returns trueage >= 18
returns true -- when the value of the variable "age" is 18 or greater.
- Comparison Operators Practice
- 4
Student Instructions
Explore Comparison Operators with Console.log
Your task: Make each expression evaluate to true by changing only the comparison operator.
We can easily test the results of comparison operators with console.log
.
- Run the code provided - see results in the console debug area below.
- All of the Boolean expressions currently evaluate to
false
. - Modify the code so they all evaluate to
true
- Change only the operator
Example:
(3 > 4)
evaluates to false...- Change it to
(3 < 4)
(3 is less than 4) to make it *true
HINT: The workspace starts in text mode because you might find that doing this in text mode is much easier and faster than dragging out new blocks. You can flip back to block mode if you like.
- Introduction to Conditionals: if Statements
- 5
Student Instructions
Understanding Program Flow
Programs are said to have a "flow of execution". You start by executing some line of code and then the next and so on.
A flow chart is a common visual that's used to represent the various paths of execution that your program might take. Many people use them to help plan.
1. This flow chart depicts a program executing one line after another until it gets to a point where it needs to make a decision.
2. In order to determine which path to take you state some condition. It should be a Boolean expression - something that evaluates to true or false. Here we have a simple comparsion of two values: the person's age and the number 18.
3. The program does one thing if the condition is true, and something else if the condition is false.
4. The program can continue a single thread of execution after the condition as well.
How If-statements work
if
statements are the lines of code you need to change the flow while you're program is running. You can write code that makes a decision that determines which lines of code should be run next.
At the right is a diagram showing the elements of a basic if
statement in JavaScript.
There are two basic parts to an if-statement.
- A condition to be evaluated (A Boolean expression that evaluates to true or false)
- Code that should run if the expression was true - enclosed in curly braces
A worked example
1.
Program executes line by line as you would expect. It displays a message, then prompts the user to enter a number. Whatever the user types will be stored in the variable age
and then proceeds to the next line...
2.
When the if
statement is encountered the first thing it does is evaluate the condition in the parentheses. It checks to see if, at this point in the program, the value in a variable age
is greater than or equal to 18. If it is then we say the expression "returns true". Otherwise it returns false.
3. These console.log statements will only execute if the expression was true. The curly braces surround all of the code that should be executed if, and only if, the expression was true. Otherwise, the entire section of code encapsulated in the if statement is skipped.
4. Execution picks up here, on the first line after the closing curly brace of the if-statement. This line will always execute, but notice that if the user entered an age less than 18, the entire if statement would be skipped, and it would just say "Thanks for verifying". (We'll fix this awkwardness soon).
Teaching Tip
Note this level cannot be validated.
Student Instructions
You try it
This is only slightly different from the voting example you just saw.
- Add an if-statement to the code to check the
age
to see if the person is old enough to drive. (In most states you need to be 16 or older). - Display a message if the person is old enough drive.
You can add an if-statement by dragging it out from the toolbox. We've provided the console.log message you should use. Just drag it inside the if-statement. This animation shows most of what you have to do.
Teaching Tip
Validation: This only checks whether the student added a call to promptNum
. It does not check whether they added an if-statement.
Student Instructions
Do it yourself - What's the secret number?
Insert lines of code between the "Welcome" and "Goodbye" that do the following:
- use
promptNum
to ask the user to enter the "secret number" - add an
if
statement to check if the number is the secret (you can make up your own secret number) - use
console.log
to display a message if the user guessed correctly
NOTE: Don't go overboard here adding messages. Just get practice adding a prompt and an if-statement on your own. Make sure it works and move on.
Student Instructions
You try it -- Text Password Checker
Key idea: You can use ==
to compare strings as well as numbers.
- Let's use an if-statement in an event handler to compare text that a user inputs in a UI element.
The setup: Note the setup in Design Mode There are two images: a red lock, and a green "unlock". The green "unlock" is hidden* at the start.
Do this:
Add an if-statement to the event handler to check the password and display the "unlock" only if the password is correct
- You can use
==
to compare the text you get from the password_input text box and a string you want to use as the password. The boolean expression you should use should look like this:
- If the user entered the correct password then show the green "unlock" and hide the red lock.
- We've provide the code to hide and show the images. You just need to add the if-statement.
- Otherwise do nothing. They can try again.
- Introduction to Conditionals: if-else Statements
- 10
Student Instructions
Points to pay attention to:
- An IF statement can have an else clause, but an ELSE clause cannot exist on its own.
- With an else clause you are guaranteeing that one of two portions of code will run.
Student Instructions
How If-Else Statements work
With an if-else statement you are giving an either-or command:
either the lines of code inside the if will execute or the lines inside the else will execute. Those are the options.
You saw in the video how to add an else clause to an if-statement -- hit the little +
symbol on the tail of the if statement.
Inside the curly braces for the else clause you put lines of code that you want to run if the Boolean condition from the if statement is false.
Some important notes about the else clause:
- The
else
must come immediately after the closing curly brace of an if statement - The
else
also has its own set of opening and closing curly braces to encapsulate lines of code
Considering our flow chart from before, until now we haven't had a way to make the program do something different if the condition was false. With an if-else
statement we do.
We can now write a program that "branches" at a particular point, running one of two possible sections of code.
A Worked Example
1. Lines of code execute sequentially as usual. Prompt the user to enter their age.
2.
The if
statement and Boolean expression are also the same as before. The expression evaluates to either true or false.
3. With an if-else
statement you are guaranteeing that exactly one of these two sections of code will execute. If the condition is true (age is 18 or greater) then the lines of code inside the if-statement's curly braces are executed. If the condition is false it jumps to the else clause and executes any lines of code it finds between the else clause's curly braces.
4. Finally the program picks up normal execution directly after the if-else
block. At this point in the program, we know that either the code in the if-block or the else block has executed.
Optional: What if you wanted to type the code instead of using blocks?
You can always type any of the code we compose in blocks. Here is an animation showing a programmer adding an else
clause to an if-statement. Notice that you can freely go back and forth between blocks and text. With text you also have a bit more leeway with formating.
In JavaScript, spaces and line breaks are the treated the same - 1 space is the same as 2 or 10 or 20.
There are certain stylistic conventions that programmers can get in heated arguments about, but there actually are no rules about style that will make your code run any differently. JavaScript looks for keywords, parentheses, curly braces and so on, but ignores extra spaces.
You usually use white space to make your code more readable.
Here are some examples of the exact same program, but formatted differently. They all work and run the same.
1.
This is the format that is used by the block-based version of code in app lab. It's trying to save on space because the blocks take up a lot of space on their own.
2.
Here is the formatting that's similar to the AP pseudocode with if
, else
, and all of the curly braces, each on their own line. This is a perfectly valid way to format code as well.
3.
Here, the programmer used line breaks to add some white space for readability. People sometimes use blank lines to separate portions of a program so that lines of code that are visually clumped together are related to each other in some way.
Student Instructions
You Try It
Recreate the simple movie age example from the video.
- We've provided the first line to prompt the user to enter their age.
- Add and
if-else
statement that checks to see if the age is>=
13 and - Use
console.log
to display appropriate messages
Here is pseudocode for what you should add
IF age >= 13 DISPLAY "You can see a PG-13 movie alone" ELSE DISPLAY "You are not old enough to see a PG-13 movie alone"
Teaching Tip
Note this level cannot be validated.
Student Instructions
You try it
Let's modify the driving age example to add an else
clause.
- Add an
else
to the if-statement. - Add a console.log message inside the else clause that says something like "sorry you can't drive yet".
You can add an else-statement by clicking on the +
attached to the if statement. We've provided some starting code.
BONUS - if they are not old enough to drive you could do the math and tell them how many years they have to wait.
Student Instructions
You try it - adding else to the password checker
If we add an else clause to the password checker we can make it act a little fancier - study the animation at right.
Add an if-else
statement to the login_btn event handler that:
- Shows the unlock and displays "Access Granted" if the user enters the password correctly.
- Otherwise, show the lock and display "Access Denied"
Note the setup:
- Again we have two images - a lock and an unlock - but this time both are hidden at the start.
- There is also a text label at the bottom where we'll display a message, but it starts empty as well
There are two event handlers
-
The first event handler - on login_btn "click" - is where you should add your
if-else
statement- We've provided the statements you need to add into the appropriate clause
-
The second event handler - on password_input "click" -- acts as a reset
- You do not need to modify anything in this event handler
- It simply hides all images and sets the message text to
""
HINT: [click to expand]
Your if-else statement is going to use the same boolean expression as before. The structure of it should look like this:
if( getText("password_input") == "some secret" ){ // show green unlock // display "Access Granted" } else { // show red lock // display "Access DENIED" }
Student Instructions
How Dropdown Menus Work
A dropdown menu provides a fixed list of choices for a user to choose from. If you're expecting only a fixed set of responses from a user then it's a good alternative to a plain text input box. For example, if you want the user to select a day of the week.
Dropdown menu v. text input
Choosing a day of the week is an example of when it's a good idea to use a dropdown menu because there are only 7 days of the week, but there are countless ways a user might type it if you used a text input box. Rather than trying to figure out what the user typed, you can provide your own list. It makes it much easier to check.
Add and populate in Design Mode
1. In Design Mode click and drag a dropdown onto the screen
2. Don't forget to give it a meaningful id
3. Add the list of options that you want here, each one on its own line. By default the options box shows only two lines, but you can add as many lines as you want.
How to get what the user chose
To get the value the user selected from the dropdown menu is very similar to getting the text out of a text input.
Design Mode
In the events tab you can click the shortcut to insert an event handler into the code.
1. The event type "change" gets triggered when the user has made a new selection. The "change" event fires when the user releases the mouse button on a new selection. (You don't want "click" because that would trigger the moment the user clicked the arrow to open up the menu in the first place.)
2.
You get the value that was selected the same way you get data out of a text input box with getText(id)
. If you call getText(id)
on a dropdown menu it returns the currently selected value in the menu.
A worked example
Here is an example that uses an if statement with a dropdown.
- The program lets the user pick a day of the week and display a message.
- Study the code and read through the commentary on the right side.
- An image of the app is shown at the left after the user has selected Wednesday.
1. When the dropdown changes
2. Save in a variable the beginning of a message that will eventually be displayed to the user: "It's <name of day>!"
3. Check the current value of the dropdown to see if it's "Friday"
4. Add text to the message. Either "Whoo hoo..." if it's Friday, or "Aw..." if it's not.
5. Finally, set the text of a label on the screen to the message that was constructed.
A note on your choices as a programmer
It's possible to write the program above without introducing the msg
variable at all. You can write it with just a single if-else
like so:
However, the programmer realized that if she wrote it like this, the code in both the if and the else would effectively contain a repeated portion:
setText("msg_label", "It's "+getText("day_dropdown")+"!...
With experience you learn that repetitious code is bad and prone to errors. In this case there are two different places that are changing a label on the screen.
If you do the same thing in two different parts of your code it means that if you want to change it, you need to do it in two places. It's easy to spot here, but in a larger program it might be tricky.
So in this case the programmer made the choice to "factor out" the repeated portion by breaking up the string into smaller parts, and making a single call to setText at the end.
Student Instructions
You Try It
This example is very similar to the worked example on the previous page - it is a version of the movie age checker that uses a dropdown.
We've provided the starting UI elements, including the dropdown - but none of the code.
Do this: Using the examples on the previous page as a guide...
- Add an event handler for the dropdown menu that triggers on "change"*.
- Add an if statement to check if the age chosen in the dropdown is
>=
to 13 and set the text in the result_label- if the age is 13 or over set the text to "You are old enough to see a PG-13 movie alone"
- Otherwise, set the text to "You are not old enough to see a PG-13 movie alone"
Tip: Use getNumber(id)
instead of getText(id)
to get the value out of the dropdown
- One major difference from the example is that we want to compare the value of the dropdown to a number using
>=
rather than with==
- You should to use
getNumber("age_dropdown")
to extract the value as a number rather than text. - This is the same reason we use
promptNum
instead ofprompt
for simple console examples. - It will still work if you use
getText
but isn't good form.
Teaching Tip
Design Note
It's important to note the inclusion of the reset()
function here...
-
We include it because to make the app function in ways that a user would expect, it turns out that you want to hide the images and clear out the last die roll when either the user makes a new selection in the dropdown or they roll the dice again.
-
However the insight to add a function was not something that occurred to us, the designers of this example, until we were in the middle of developing it. It came about organically -- as we were developing this example we realized we needed to repeat code in two different places.
-
It's therefore a perfect example of when to introduce a function - you want the same code to be able to be called from different parts of your code, at different times.
What that means for students:
- They should not "feel bad" if they are worried that they don't understand where this function came from or about their ability to think of such things
- But they should able to understand the code, trace what's happening with the function, and understand why, in the end, it is there.
- We left it in because it's a good example of when one should write a function.
Student Instructions
You try it
This is a slightly more involved example. You'll need to study the starting design and code a little bit, but you only have to insert a single if-else statement and move some lines of code into the appropriate if or else clause to make it work.
Do this
Study the starting code first to see what it does
- You can run it to see what happens before doing anything - it just won't work right
- Notice the function
reset()
- which hides the images.- We made a function because we want to run this code from two different event handlers.
Add an if-else statement in the roll_btn event handler to check if the random number that represents a dice roll matches the user's guess in the dropdown
If they guessed correctly Show the trophy image Add 6 to the score
Otherwise: Show the frowny face image Subtract 1 from the score
Setup Notes:
All UI elements are added for you (images are hidden) - flip to Design Mode to see.
All lines of code except for the if-else statement and condition are also provided - you'll need to move them around once you've added the if-else statement.
Notice the global score
variable which will keep track of the score between clicks
We've added comments in the code to help you out - you can remove them if you like.
Hint: [click to expand]
The condition needs to us
==
compare dieVal
with the value in the dropdown. To get the value out of the dropdown you use getNumber("guess_dropdown")
.(You could use
getText("guess_dropdown")
and it would still work, because ==
tries to convert text to a number for comparison so "6"==6
is true. But since we're expecting a number in the dropdown we should use getNumber
so it makes sense when reading the code.)
Teaching Tip
Validation Note: This level checks only for the presence of:
an event handler - supposed to be for the dropdown menu on "change"
a call to getText
- presumably to get the text out of the dropdown menu they are supposed to add
a call to setText
- presumably to display the appropriate message in the label with id: msg_label*
Teaching Tips:
-
Students may discover the
if-else-if
construct here because it's easy to find by simply clicking on the+
next to the else clause. -
However, the
if-else-if
structure is what you're learning next and this challenge is meant to motivate the need for it. Up to you whether you want to force students to use nestedif
statements here or not. -
One reason to use nested statement is that that's what you'll see on the AP exam. The AP pseduocode has no concept of the
if-else-if
shorthand. So it's good practice for that.
Student Instructions
Challenge: It's the weekend!
Make an app tells you whether to stay home or go to school based on what day of the week it is.
In this challenge you will:
- Add your own dropdown menu in design mode
- Add an event handler for the dropdown
- Write an if-statement that's slightly tricky
Do this:
- In Design Mode add a dropdown menu and fill it with the days of the week: Monday, Tuesday,...,Sunday
- Add an event handler for that dropdown that activates on "change"
-
Add an
if-else
statement to the event handler that checks which day was selected and...-
If the day selected was a weekend day (Saturday or Sunday) then display a message that says "It's the weekend! Stay home!"
-
Otherwise (the day is a weekday) display a message that says "It's a weekday. Go to school".
- Your message should be displayed by setting the text of the msg_label provided in design mode.
-
Testing:
- Once you have something functional, make sure you test every day of the week to verify the output is correct
- The if-statement here is trickier than before.
HINT: [click to expand]
IF it's saturday ... ELSE IF it's sunday ... ELSE ...
Standards Alignment
View full course alignment
CSTA K-12 Computer Science Standards (2011)
CL - Collaboration
- CL.L2:3 - Collaborate with peers, experts and others using collaborative practices such as pair programming, working in project teams and participating in-group active learning activities.
CPP - Computing Practice & Programming
- CPP.L2:5 - Implement problem solutions using a programming language, including: looping behavior, conditional statements, logic, expressions, variables and functions.
CT - Computational Thinking
- CT.L2:1 - Use the basic steps in algorithmic problem-solving to design solutions (e.g., problem statement and exploration, examination of sample instances, design, implementing a solution, testing and evaluation).
- CT.L2:14 - Examine connections between elements of mathematics and computer science including binary numbers, logic, sets and functions.
- CT.L2:3 - Define an algorithm as a sequence of instructions that can be processed by a computer.
- CT.L2:6 - Describe and analyze a sequence of instructions being followed (e.g., describe a character’s behavior in a video game as driven by rules and algorithms).
- CT.L2:8 - Use visual representations of problem states, structures and data (e.g., graphs, charts, network diagrams, flowcharts).
Computer Science Principles
4.1 - Algorithms are precise sequences of instructions for processes that can be executed by a computer and are implemented using programming languages.
4.1.1 - Develop an algorithm for implementation in a program. [P2]
- 4.1.1A - Sequencing, selection, and iteration are building blocks of algorithms.
- 4.1.1B - Sequencing is the application of each step of an algorithm in the order in which the statements are given.
- 4.1.1C - Selection uses a Boolean condition to determine which of two parts of an algorithm is used.
5.5 - Programming uses mathematical and logical concepts.
5.5.1 - Employ appropriate mathematical and logical concepts in programming. [P1]
- 5.5.1E - Logical concepts and Boolean algebra are fundamental to programming.
- 5.5.1G - Intuitive and formal reasoning about program components using Boolean concepts helps in developing correct programs.
CSTA K-12 Computer Science Standards (2017)
AP - Algorithms & Programming
- 2-AP-12 - Design and iteratively develop programs that combine control structures, including nested loops and compound conditionals.
- 3A-AP-15 - Justify the selection of specific control structures when tradeoffs involve implementation, readability, and program performance and explain the benefits and drawbacks of choices made.