Lesson 5: Building an App: Clicker Game
Overview
In this lesson, students add variables to two different exemplar apps to keep track of a score, or a count of some number of button clicks. The major topic is variable scope and understanding the differences, benefits, and drawbacks, of using global versus local variables. This lesson focuses more on using global variables, since in event-driven apps that’s what you need to keep track of data across multiple events.
The very basics of a simple if statement are also presented in this lesson, mostly to highlight the difference between the = and == operators. Finally, students are asked to apply what they’ve learned about variables, scope, and if statements, to make their own “clicker” game modeled after one of the exemplars they saw during the lesson.
Purpose
This lesson is mostly a continuation and furthering of our understanding of variables and how they work. There are many, many pitfalls and misconceptions about variables and how to use them in programs for the early learner. Variables are often difficult to learn because they are not visual, they are abstract, and one must have a good mental model for what’s happening in the computer and with program instructions, in order to reason about the code and develop one’s own solutions to problems.
The topic and concept of variable scope is a big one in any programming language. However, since many languages do it differently, the concept of variable scope isn’t listed explicitly as a learning objective in the CSP framework. As a concept, though, variable scoping is a form of abstraction - a programming language lets you create variables with as narrow or broad a scope as you need to program a certain task. As a general practice, you usually want to create variables with the most narrow scope you can for the task at hand, since the other extreme - everything is global - can become unwieldy or hard to manage, and it doesn’t promote code reuse.
Agenda
Getting Started (5 Minutes)
Activity (90 Minutes)
Wrap-up (10 Minutes)
View on Code Studio
Objectives
Students will be able to:
- Use global variables to track numeric data in an app.
- Give a high-level explanation of what “variable scope” means.
- Debug problems related to variable scoping issues.
- Modify existing programs to add and update variables to track information.
- Create a multi screen "clicker" game from scratch
Preparation
- Decide whether you want to introduce the activity guide at the beginning of the lesson or the end.
- Familiarize yourself with the Clicker Game and rubric, decide how to organize peer review.
Links
Heads Up! Please make a copy of any documents you plan to share with students.
For the Students
- Activity Guide - The Clicker Game - Activity Guide
Vocabulary
- == - The equality operator (sometimes read: "equal equal") is used to compare two values, and returns a Boolean (true/false). Avoid confusion with the assignment operator "=",
- Global Variable - A variable whose scope is "global" to the program, it can be used and updated by any part of the code. Its global scope is typically derived from the variable being declared (created) outside of any function, object, or method.
- If-Statement - The common programming structure that implements "conditional statements".
- Local Variable - A variable with local scope is one that can only be seen, used and updated by code within the same scope. Typically this means the variable was declared (created) inside a function -- includes function parameter variables.
- Variable Scope - dictates what portions of the code can "see" or use a variable, typically derived from where the variable was first created. (See Global v. Local)
Introduced Code
Teaching Guide
Getting Started (5 Minutes)
Recall basic mechanics and terminology of working with variables
Recall In the previous lesson, we learned about the basic mechanics of working with variables in JavaScript. We developed a mental model for thinking about how values are stored and retrieved from memory and that we should read the “=” sign as “gets” to avoid confusion.
Moving forward The whole purpose of learning about variables though is so that our apps can make use of them while a program is running. In this lesson, we’ll see how to do that. So let’s get to it.
Activity (90 Minutes)
App Lab: Building an App - Clicker Game
Teacher Note:
- This Activity Guide - The Clicker Game - Activity Guide is not strictly needed until the very end of the lesson -- it is referred to in level 21
- However, if you think it would provide some motivation, you may want to optionally show this activity guide at the beginning of the lesson.
Wrap-up (10 Minutes)
Peer Review of Clicker Games
Activity Guide - The Clicker Game - Activity Guide contains a rubric that students can use to evaluate their classmates’ apps. It is up to you to determine who should evaluate which programs, how to pair or group students, and the degree of anonymity you wish to maintain.
Peer review should be a useful activity for students in preparation for the Create Performance Task in which they need to give and receive feedback with a partner to improve their programs.
Transition: Now that we understand a bit about variables and how to use them in our programs, a whole new world will open to us. First, we will learn that variables can hold other kinds of data besides numbers. We’ll also learn other ways to get data from the user using other UI elements like text input, pull-down menus, and so forth.
- Lesson Overview
- Student Overview
- Clicker Game Demonstration
- 2
Student Instructions
Clicker Game Demo
Try out this clicker game! You'll be building your own version (with a theme you choose) at the end of the lesson.
Things That Are Familiar
You know how to build many of the components of this game already: - Multiple screens. - Buttons that change the screen when clicked. - Image that moves when you click it.
Things That Are New
- Keeping track of and displaying the changing score.
- Keeping track of and displaying the changing lives.
- Changing to the win screen if the score is 25.
- Changing to the lose screen if the lives is 0.
Student Instructions
Changing Elements on Screen
There's another way to display text in your app besides console.log
and write
.
There is a command called setText
which will change the text of a component on screen given its id. This is a very powerful technique and one that you will use a lot. Here's what it looks like in action....
Let's do a very simple example of using setText
.
Do This:
* Run the code in the app.
Look at the code for the "upArrow" event handler and how it sets the text of the label.
- Modify the code.
When the down arrow is clicked, set the text of the label to something else.
- GOAL:
- Your only goal is to successfully use
setText
in this example app. - The app should do something similar to the animated example at right.
Once you've got it functional, click Finish to move on.
Student Instructions
All the Basics You Need
When you close these instructions, you'll get to play a little bit with the very simple app (shown at right) to demonstrate the basics of concepts involved in making the clicker game. We'll call it the Count Up/Down App. Yes, it's a boring "game," but it has all the pieces of a more sophisticated game.
This app demontrates a few new concepts. We'll step through each one, one at a time, explaining how each thing works. Along the way, we'll also encounter some common challenges, and learn how to solve them.
Do This:
- Keeping clicking the up arrow until something happens (something besides the number increasing).
- Start over.
- Click the down arrow until something happens (something besides the number decreasing).
- Start over.
Once you think you've seen all the behavior click Finish to see the first part of how this is done.
Student Instructions
Using Variables in Apps!
The example program here has a small portion of the Count Up/Down App written. Right now, what the app should do is shown in the animation to the right.
Important Concept!
This small functionality demonstrates an important concept - how to create a variable in the app and update it when an event occurs. It may seem fairly straightforward but there are some common misconceptions that we want to alert you to.
Do This: Misconception Investigation
Over the next few levels you are going to do a small investigation of two apps that do almost the same thing but one works as expected and one with a bug that demonstrates an important concept about using variables in apps. Here is what you'll do:
- Run this app, which works properly.
- Study the code until you think you understand what's happening.
-
Pay attention to the code that handles the up arrow being clicked.
-
Run the app on the next screen, which has a bug.
- On the next screen we show you almost the same app, but with a subtle problem.
-
See if you can spot the difference and fix it.
-
Report what you found!
- We'll ask you to report what you found. It's not a quiz. You can go back and forth until you spot the problem, but you should find it and be able to write what it is.
Student Instructions
Debugging Problem!
Uh oh! The code here is subtly different from the one in the previous example and now there is a problem.
Do This:
- Run the app and try it to see the problem.
- See the error.
- Note that NaN stands for "Not a Number" - why would it say this?
- Note the difference between this broken version and the previous one that worked.
- Fix the problem so that it works as before.
- Reflection on Debugging
- 7
Student Instructions
Respond to the following questions about your investigation into the bug with the counting game. Feel free to go back and look at the game again (but do so in another tab or be sure to click "Submit" to save your work!):
- From the user's perspective, what was the difference between the first version of the game and the one with the bug?
- From looking at the code, what specifically was the difference between the two, and why is that an issue?
- How did you fix the bug?
- Variable Scope: Local vs. Global
- 8
Teaching Tip
This level is a lengthy (but necessary) description of variable scope.
While variable scope isn't explicitly mentioned in the CSP framework, it is basically impossible to program in any programming language without understanding this concept.
Please make sure that students read this, or that you review it as a class
Student Instructions
Creating Variables in the Right Place
td{ width: 400px; vertical-align: top; }
Problem: writing var count again
The problem you encountered was caused by Line 4: |
![]() |
|
|
Solution: remove var from Line 4
Instead of trying to create a new variable inside the event handler, this code will now reference the one created in Line 1. Because we only created the variable once, all references to |
![]() |
Where You Decide to Create Variables in Your Code Matters
In Javascript you have two choices:
-
Create a variable outside of any function.
-
Create a variable inside a function.
The term for this concept is "variable scope." The scope of a variable defines which parts of your code can access and modify the variable.
Understanding Variable Scope
Javascript has two scopes: global and local. Here's the difference...
Local Variables
Variables created within a function become local to the function. Local variables have local scope: they can only be accessed within the function. In the example to the right we say that age is "local to" someFunction which means that only code within someFunction can access and modify the value age. Code that's outside this function cannot see or use the variable. In fact, local variables are created when a function starts, and destroyed when the function is completed. Local variables only exist for the life of one function call. |
![]() |
Global Variables
A variable declared outside a function, becomes global. A global variable has global scope which means the variable is accessible and modifiable throughout your program by any code, and also in any function.
In the example to the right we say that lives is a global variable. |
![]() |
Why Use Local Variables?
Local variables are useful temporary placeholders for data that you need to perform a computation of some kind.
Often a program might have several functions that perform different but related tasks, and you'd like to reuse variable names. Since local variables are only recognized inside their functions, variables with the same name can be reused in different functions.
The best example of this is function parameters. Function parameters are a form of local variable - they get created and initialized when a function is called, they get used while the function runs, and then they get destroyed when the function completes.
Imagine if all we had were global variables. Then you could not write this code:
If all variables had to be global we'd have to invent different variable names for every function parameter in the entire program. But because the size variable is local to the function, we can re-use this handy parameter name.
Common Pitfalls with Local Variables
-
The most common mistake is something you just experienced: accidentally re-creating a variable inside a function when you intended to reference a global variable.
-
When you create a variable inside a function, and it happens to have the same name as a global variable, the local variable takes precedence and the function will try to use the local variable first.
Why Use Global Variables?
Global variables are useful for keeping track of data over the lifetime of the program that's running.
If you want to keep track of some data between events, or between function calls, you want a global variable.
Where to Create Global Variables
By convention global variables are created and initialized in the very first lines of code in a program.
Common Pitfalls with Global Variables
-
Because you can create a global variable anywhere in your code that is outside of a function definition, you can get yourself into trouble if you have variable declarations scattered throughout a large program. You might accidently re-create or create a new variable for a purpose you've already made a variable for.
-
ADVICE: Stick to the convention: if you need a global variable in your program, create it and give it an initial value at the very top of your program. Keep all global variables in a group at the top where you can look them up.
Moving On...
Now let's practice a little bit more with variables.
For our programs coming up we're particularly interested in global variables since that gives us the ability to remember data like the score of a game over the life of a program.
Student Instructions
Make Count Down Work
You now know enough about using global variables (and some of the problems you might run into) to try it yourself.
Let's start with an easy task. Right now clicking the up arrow works as expected; clicking the down arrow does not. So...
Do This:
Make the count go down by 1 every time the down arrow is clicked
- Study how the up arrow works, and use it as an example for writing the code for the down arrow.
- Add and modify the code so that when the down arrow is clicked the count goes down.
- When you're done the app should work like the animation shown to the right.
- HINT: Don't forget to set the text of the label.
Student Instructions
Bug Squash!
This program has a bug that doesn't produce an error. It just doesn't do what's expected. The reason is a common mistake that all programmers make.
Do This:
- Run the program.
- To see the bug:
- Click the up arrow about 5 times.
- Click the down arrow about 10 times.
- Click the up arrow again.
- Look at the code and fix the problem.
- Read about the common mistake by expanding the area below.
- Once you've fixed the issue, move on.
Read about the common mistake here... [click to expand]
...forgetting to update the display after changing something in the programMisconception Alert - Changing a Variable Doesn't Change the Display
A common misunderstanding about variables and displaying them is to think that a text label that's displaying a variable will change when the variable changes. NO. A text label is just "dumb" container for text. It's similar to a variable itself in that it won't change unless you explicitly tell it to.
Concept: Separation of Program Data from How It's Viewed
Maintaining variables and program data is a different task from maintaining the display of the app. Your program could actually run without updating the display at all - it would still be working behind the scenes; it just wouldn't be very fun or interesting to use.Student Instructions
Bug Squash!
This program has a few different bugs that are related to the same problem.
Do This:
- Run the program.
- To see the bug:
- Click the up arrow several times.
- Click the down arrow.
- Look at the code and fix the problem.
- Read about the common mistake by expanding the area below.
- Once you've fixed the issue, move on.
Read about the common mistake here... [click to expand]
...thinking you're referencing a global variable when you're not.A common mistake is basically a syntax/spelling error. These mistakes can be really tricky to work out because you think you know what you wrote, but the computer doesn't see it that way :)
Student Instructions
Bug Squash!
This program has a few different bugs. Find them and squash them!
Do This:
- Run the program.
- To see the bug:
- Click the up arrow exactly twice.
- Restart the program and do this a few times - you'll notice nothing happens the first time you click.
- Click the down arrow several times.
- Look at the code and fix the problem.
- Read about the common mistake by expanding the area below.
- Once you've fixed the issue, move on.
Read about the common mistake here... [click to expand]
...updating the wrong thing in the wrong event handler. There are two common mistakes here: 1. Mixing up which event handler should do what. 2. Updating the display before any change is made to the underlying data of the program. This can make the app seem oddly out of sync where each event triggers an update to the display that reflects the last thing done, not the current thing.Student Instructions
Using Global Variables
You'll now look at a version of the clicker game. We've set up the basic functionality to move the apple around the screen, and have created a global variable to keep track of the score.
Do This:
Add code to update the score when the apple is clicked. Remember that you'll have to update both the global variable and the label text!
Student Instructions
Tracking Lives
In the game, the number of lives starts at 3 and decrements by 1 every time the background image is clicked. Add this functionality to your game!
Do This:
- Add your variable to keep track of lives.
- Add a click handler for the background image. ID: "background"
- When the background is clicked, decrement the number of lives by 1.
Teaching Tip
Teacher Note - We're not teaching if statements...yet
While there is a lot of text here this is NOT actually where we learn formally about if
statements. That comes in the next set of lessons.
We simply want the game to be able to end, and for that we need a simple if
statement that hopefully won't stretch students too far.
All that's needed here is enough understanding to write a simple if
statement to end the game. Like this:
if (score == 20){ setScreen(...); }
So if students get hung up on this page just encourage them to move on.
Student Instructions
Having Your App Make Decisions
Now that you have variables keeping track of useful information in an app, the next thing you probably want to do is make decisions based on their values.
For example: in the Count Up/Down App what we want to say is "if the count reaches 20 then switch screens"
NOTE: we're going to learn all about if
statements later on. For now, we need just enough to make our program end. If you can understand enough to copy this example then you're good.
Add an if-statement to the event handler
We want to add an if
statement into the event handler to check the value of count
immediately after we update its value. Here's what the full event handler should look like:
Notice: use ==
to compare values. As you know the simple =
means assign a value to a variable. So we need a different operator to check if one value is equal to another. That operator is the "equal equal" operator: ==
.
More Explanation Below This Line
If you understand the example above and feel like you could incorporate that into the Count Up/Down app, then you you can probably continue without difficulty. You can always come back to this page if you want to read the more complete explanations.
if Statements
Most programming languages have a way to do this: to check to see if some condition is true, and if it is do something. It's called an "if
statement". if
statements are a big topic in and of themselves, but for now we'll just look at a simple example to meet our current needs.
Here's an example of an if
statement (shown in both block and text form)
![]() |
![]() |
There are a couple of things happening here that we should explain.
How to Add an if Statement to Your Program
In the toolbox you'll now see an if
statement. To add it to your program just drag it out. For our current program we want to check the value of count inside the up arrow event handler.
You'll notice it has an empty space where you need to insert the condition that you want to check. We'll get to that next.
The if
statement defines a block of code to execute between open and closing curly braces {}.
If the condition is true then the block of code inside the curly braces is executed from top to bottom, exactly once. If the condition is false then the entire block of code is ignored.
The Operators == vs. =
=
read as: "gets"
==
read as: "equal-equal"
The reason why we recommended that you read =
as "gets" is really to avoid confusion with the ==
operator, which to avoid confusion even further, many programmers read as "equal-equal".
To state what should be obvious by this point: =
is for assigning a value to a variable and ==
is for checking or testing to see what a value is. You can probably imagine the number of mistakes that beginners might make here.
TIP: if you continue to read code "aloud" in your head using "gets" and "equal-equal" it might help you discover bugs in your code or avoid them in the first place. For example, here is an arbitrary piece of code:
code | read as |
---|---|
![]() |
"var a gets 7. If a equal-equal 9 then a gets 0" |
Misconception Alert - Where to Put if Statements
An if
statement does not constantly monitor your program checking the condition to see if it's true or false. An if
statement is an instruction just like any other that gets executed line by line in order from top to bottom.
This means that you need to insert an if
statement into your code at the exact place where you need the condition checked. Once the condition is checked program execution picks up at the end of the if
statement and proceeds from there.
This is why we need to check the value of count
EVERY time the up arrow is clicked. So that means the if
statment must be written into the event handler for the up arrow, and checked after we update the value of count
. Here's what the full event handler should look like:
Student Instructions
Add Your Own if Statement
In most apps you want to make decisions based on the state of some data you're keeping track of in the app.
We've modified the Count Up/Down App to add another screen. When the count reaches certain values, we'll switch screens. In the code you'll see an if
statement has been added to the event handler for up arrow that states:
- If the value of
count
is equal to 20, then set the screen to "gameOverScreen".
Do This:
Add an if
statement so that when counting down the app changes screens when the count reaches -5.
- Run the app and click the up arrow 20 times to see what happens when the
if
statement is triggered. - Study the
if
statement for the up arrow button to see how it works. - Add an
if
statement to the down arrow event handler:- If
count
is equal to -5, then set the screen to the "gameOverScreen."
- If
- Goal: Once you're finished, clicking the down arrow in the app should work like the animation shown to the right.
HINT: [click to expand]
There is a subtle challenge here that you need to set the text of the label on the gameOverScreen when you change screens.NOTE: Your code can call
setText
for any UI element, on any screen, at any time - even if the element isn't on the screen that's currently showing.
Student Instructions
Add a Reset Button
In most apps you want to provide a way to start over.
To do this without having the user quit and restart your app, you need to add code to reset variables and text displays to initial values so the app can effectively start over.
We've added a button to the "gameOverScreen" in the Count Up/Down App and added an empty event handler for it.
Right now clicking the "start over" button does nothing. You will write code to make it work.
Do This:
Add code to the startOverButton event handler to reset the app.
The app should work like the animation shown to the right. Notice that when the "start over" button is clicked it goes back to the main screen and the count has been reset to 0.
The code should:
- Set the screen to the gamePlayScreen.
- Set the count variable to 0.
- Set the correct text label on the gamePlayScreen to show the count is 0.
Once you get it to work, move on.
Student Instructions
Bug Squash!
The Count Up/Down App has a bug!
A very common mistake has been introduced into the code. It's one that vexes early programmers, but we're sure you'll find it.
Do This:
-
Run the program.
-
To see the bug:
- Click the up arrow just once.
- Restart the program.
-
Click the down arrow just once.
-
Look at the code and fix the problem.
-
Read about the common mistake by expanding the area below.
-
Once you've fixed the issue, move on.
Read about the common mistake here... [click to expand]
...using
=
instead of ==
Yup, we told you this is a common mistake! And it's an easy one to make.
Remember that the single =
sign does assignment and it actually also evaluates to true. This means that if you stick it in an if
statement, that if
statement will always be true.
One strategy to avoid this mistake is to read code aloud in your head and don't even use the single word "equal":
=
==
If you get in the habit of thinking that way, these mistakes are easier to catch. For example you'd see this:
if (count = 20)
and read:
"if count gets 20" ...and know that that doesn't make sense.
Student Instructions
Bug Squash!
The Count Up/Down App has a bug!
A common mistake has been introduced into the code.
It's a tricky one to find because at first it looks like everything is okay.
Do This:
-
Run the program.
-
To see the bug:
- Click the up or down arrow until you get to the "gameOverScreen."
- Click "start over."
-
Click the up or down arrow again... what the?
-
Look at the code and fix the problem.
-
Read about the common mistake by expanding the area below.
-
Once you've fixed the issue, move on.
Read about the common mistake here... [click to expand]
...forgetting to reset everything you need to actually start over.
Frequently to actually reset you need to set a few variables back to initial values and update all the UI components, especially those that rely on those variables, so they reflect the new values.
Another common mistake shown here is setting the text of a label to "0" rather than the value of the count variable. For example, these two lines of code are a little dangerous:
count = 0; setText("countDisplayLabel", 0);
To be safe, if a label is supposed to display the value of a variable, you should always use the variable instead of hard-coding numbers as a check on yourself. The general rule of thumb is: never hard-code a value instead of using a variable that holds the value you need to show.
A common strategy for handling this is to put everything you need to reset the app into a function which you can call at both the beginning of your program, and from other screens later on. For example:
function resetAll(){ count = 0; setText("countDisplayLabel", count); setScreen("gamePlayScreen"); }
Then in some other code like a button event handler you can just call your reset
function:
onEvent("startOverButton", "click",
function() {
resetAll();
});
Student Instructions
Bug Squash!
The Count Up/Down App has a bug!
A common mistake has been introduced into the code.
We've changed the app so that it counts up and down by 3 rather than by 1.
Do This:
-
Run the program.
-
To see the bug:
- Click the up or down arrow trying to get to the game over screen.
-
It should be impossible to get to the game over screen.
-
Look at the code and fix the problem.
-
Read about the common mistake by expanding the area below.
-
Once you've fixed the issue, move on.
Read about the common mistake here... [click to expand]
...the condition you're checking in your
if
statement is actually impossible to reach.
This problem was probably easy to see here, but in practice logic errors like this can be devilish to track down. It's especially hard because the program gives you no hints that anything is wrong - it is syntactially a correct program. The computer cannot tell ahead of time whether your if
statements will ever be true. So you need to trace through the logic of your program step by step to try to figure out why something's not happening that you expect should have happened.
- Project: Clicker App
- 21
Student Instructions
Make Your Own "Clicker" Game
You will be creating your own “clicker” game similar to the Apple Grabber game you worked on in this lesson. The general object of the game is to click on an element that jumps around every time you click it. You will pick your own theme and decide what the rules are and how to keep score.
Your Main Tasks Are To:
- Pick a theme for your game and add appropriate images and styling.
- Add variables to track some data during gameplay.
- Add code to event handlers to update the variables and display appropriately.
See Activity Guide for Requirements
There is a full activity guide and rubric for this project. You can find a link to it in the student resources section for this lesson. Or ask your teacher for it.
Template
This level is a template for the app. You should run it to see what it does right now. You will modify this template, both the design elements and the code, for your project.
The template has 4 screens and some basic navigation functionality and event handlers set up for you. The game play screen uses the images from the Apple Grabber game, but you should replace these with images related to your chosen theme.
- AP Practice Response - Choosing an Abstraction
- 22
Student Instructions
AP Practice - Create PT - Choosing an Abstraction
One component of the AP Create Performance Task is creating and describing an abstraction in your program. Here's the actual prompt.
-
2d. Capture and paste a program code segment that contains an abstraction you developed individually on your own (marked with a rectangle in section 3 below). This abstraction must integrate mathematical and logical concepts.
Explain how your abstraction helped manage the complexity of your program.
(Must not exceed 200 words)
Here's one row of the scoring guide for this question
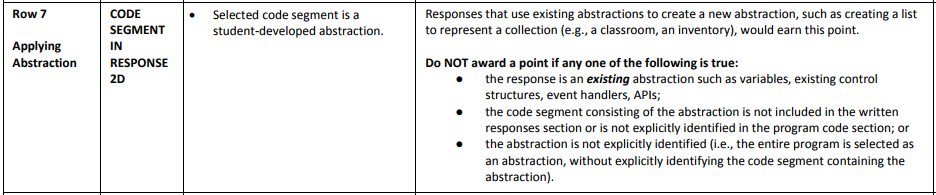
Grade the Response
Below is a segment of code from a "clicker" program with a rectangle drawn around a portion of the code identifying an abstraction.
Each row is worth one point that either can or cannot be awarded. Explain why you would or would NOT award the point for Row 7 based on the criteria given above.
Standards Alignment
View full course alignment
CSTA K-12 Computer Science Standards (2011)
CL - Collaboration
- CL.L2:4 - Exhibit dispositions necessary for collaboration: providing useful feedback, integrating feedback, understanding and accepting multiple perspectives, socialization.
CPP - Computing Practice & Programming
- CPP.L2:5 - Implement problem solutions using a programming language, including: looping behavior, conditional statements, logic, expressions, variables and functions.
- CPP.L3A:3 - Use various debugging and testing methods to ensure program correctness (e.g., test cases, unit testing, white box, black box, integration testing)
- CPP.L3A:4 - Apply analysis, design, and implementation techniques to solve problems (e.g., use one or more software lifecycle models).
- CPP.L3A:5 - Use Application Program Interfaces (APIs) and libraries to facilitate programming solutions.
CT - Computational Thinking
- CT.L2:12 - Use abstraction to decompose a problem into sub problems.
- CT.L3A:1 - Use predefined functions and parameters, classes and methods to divide a complex problem into simpler parts.
- CT.L3A:3 - Explain how sequence, selection, iteration, and recursion are building blocks of algorithms.
Computer Science Principles
1.1 - Creative development can be an essential process for creating computational artifacts.
1.1.1 - Apply a creative development process when creating computational artifacts. [P2]
- 1.1.1A - A creative process in the development of a computational artifact can include, but is not limited to, employing nontraditional, nonprescribed techniques; the use of novel combinations of artifacts, tools, and techniques; and the exploration of personal cu
- 1.1.1B - Creating computational artifacts employs an iterative and often exploratory process to translate ideas into tangible form.
1.2 - Computing enables people to use creative development processes to create computational artifacts for creative expression or to solve a problem.
1.2.1 - Create a computational artifact for creative expression. [P2]
- 1.2.1A - A computational artifact is anything created by a human using a computer and can be, but is not limited to, a program, an image, audio, video, a presentation, or a web page file.
- 1.2.1B - Creating computational artifacts requires understanding and using software tools and services.
- 1.2.1C - Computing tools and techniques are used to create computational artifacts and can include, but are not limited to, programming IDEs, spreadsheets, 3D printers, or text editors.
- 1.2.1D - A creatively developed computational artifact can be created by using nontraditional, nonprescribed computing techniques.
- 1.2.1E - Creative expressions in a computational artifact can reflect personal expressions of ideas or interests.
1.2.3 - Create a new computational artifact by combining or modifying existing artifacts. [P2]
- 1.2.3A - Creating computational artifacts can be done by combining and modifying existing artifacts or by creating new artifacts.
5.1 - Programs can be developed for creative expression, to satisfy personal curiosity, to create new knowledge, or to solve problems (to help people, organizations, or society).
5.1.1 - Develop a program for creative expression, to satisfy personal curiosity, or to create new knowledge. [P2]
- 5.1.1A - Programs are developed and used in a variety of ways by a wide range of people depending on the goals of the programmer.
- 5.1.1B - Programs developed for creative expression, to satisfy personal curiosity, or to create new knowledge may have visual, audible, or tactile inputs and outputs.
- 5.1.1C - Programs developed for creative expression, to satisfy personal curiosity, or to create new knowledge may be developed with different standards or methods than programs developed for widespread distribution.
5.2 - People write programs to execute algorithms.
5.2.1 - Explain how programs implement algorithms. [P3]
- 5.2.1C - Program instructions may involve variables that are initialized and updated, read, and written
5.4 - Programs are developed, maintained, and used by people for different purposes.
5.4.1 - Evaluate the correctness of a program. [P4]
- 5.4.1C - Meaningful names for variables and procedures help people better understand programs.
5.5 - Programming uses mathematical and logical concepts.
5.5.1 - Employ appropriate mathematical and logical concepts in programming. [P1]
- 5.5.1D - Mathematical expressions using arithmetic operators are part of most programming languages.
CSTA K-12 Computer Science Standards (2017)
AP - Algorithms & Programming
- 2-AP-11 - Create clearly named variables that represent different data types and perform operations on their values.
- 3A-AP-16 - Design and iteratively develop computational artifacts for practical intent, personal expression, or to address a societal issue by using events to initiate instructions.